Python notes
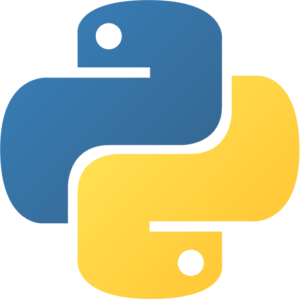
Collection of random notes and snippets I’ve needed to look up too often.
These are not in any particular order or arranged anyhow.
Most of these are joinked from Stackoverflow.
Create and write into a file
To write to an existing file, you must add a parameter to the open()
function:
"a"
– Append – will append to the end of the file
"w"
– Write – will overwrite any existing content
Open the file “demofile2.txt” and append content to the file:
f = open("demofile2.txt", "a")
f.write("Now the file has more content!")
f.close()
#open and read the file after the appending:
f = open("demofile2.txt", "r")
print(f.read())
Open the file “demofile3.txt” and overwrite the content:
f = open("demofile3.txt", "w")
f.write("Woops! I have deleted the content!")
f.close()
#open and read the file after the appending:
f = open("demofile3.txt", "r")
print(f.read())
For loop over files in a directory
for filename in os.scandir(path):
...
Check for specific file extension
if filename.name.endswith('.dmp'):
...
Variable as function name
globals()['use_variable_as_function_name']()
is equivalent to:
use_variable_as_function_name()
Print variable (int) with string
print("modified contents of " + str(fileCount) + " files.")
Read file into variable
with open(filename, 'r') as file:
var = file.read()
file.close()
for loop number range
for i in range(0,10):
print("i is", i)
Adding characters to string
Strings are immutable so you can’t insert characters into an existing string. You have to create a new string.
text = "Value"
text_new = "d" + text
print(text_new)
Output:
dValue
Capitalize a string
text = "cat"
print(text.capitalize())
Output:
Cat
Dictionaries
Python dict:
thisdict = {
"brand": "Ford",
"model": "Mustang",
"year": 1964
}
print(thisdict)
Output:
{'brand': 'Ford', 'model': 'Mustang', 'year': 1964}
Iterate over keys of a dict:
for item in thisdict.keys():
print(item)
Output:
brand
model
year
Same for values:
for item in thisdict.values():
print(item)
Output:
Ford
Mustang
1964
Iterate and enumerate list
stuff = ["Milk", "Eggs", "Corn", "Dirt", "Stone", "Banana", "Car"]
for idx, item in enumerate(stuff):
print(idx, item)
Output:
0 Milk
1 Eggs
2 Corn
3 Dirt
4 Stone
5 Banana
6 Car
Check if variable is in list
sample = ['one', 'two', 'three', 'four']
var = "four"
if var in sample:
print("four is in list")
Check if any of wanted characters is in string
s = "Hello Kebab"
chars = set('0123456789$,')
if any((c in chars) for c in s):
print('Found')
else:
print('Not Found')
Split string from character
s = "www.example.com"
parsed = s.split('.')
print(parsed)
Produces a list
['www', 'example', 'com']
Take input arguments and use them
An example snippet that takes two arguments, inputfile and onputfile, and then parses these. You can then add functionality into the TODO section.
#!/usr/bin/pythons
import sys
import getopt
import os
def second(inputfile, outputfile):
if (inputfile == ""):
print("Inputfile was not given")
else:
print ('Inputfile is: ', inputfile)
if (outputfile == ""):
print("Outputfile was not given")
else:
print ('Outputfile is: ', outputfile)
#TODO: Add functionlity here or call another function
def main(argv):
inputfile = ''
outputfile = ''
try:
opts, args = getopt.getopt(argv,"hi:o:",["ifile=","ofile="])
except getopt.GetoptError:
print ('python3 playerHelper.py -i <inputfile> -o <outputfile>')
sys.exit(2)
for opt, arg in opts:
if opt == '-h':
print ('python3 playerHelper.py -i <inputfile> -o <outputfile>')
sys.exit()
elif opt in ("-i", "--ifile"):
inputfile = arg
elif opt in ("-o", "--ofile"):
outputfile = arg
second(inputfile, outputfile)
if __name__ == "__main__":
main(sys.argv[1:])
Example usages.
input:python3 playerHelper.py -h
results:python3 playerHelper.py -i <inputfile> -o <outputfile>
input:python3 playerHelper.py
results:Inputfile was not given
Outputfile was not given
input:python3 playerHelper.py -i ayy.txt -o brrr.txt
results:Inputfile is: ayy.txt
Outputfile is: brrr.txt